Mobile Device Features 2: Difference between revisions
Jump to navigation
Jump to search
Line 29: | Line 29: | ||
= Geolocation = | = Geolocation = | ||
[[geolocation.jpg]] | |||
* Geolocation uses GPS sensors to return the current location of the device. | |||
* The watchPosition() function sets the frequency of the Geolocation events. | |||
* When a Geolocation event is triggered, the function named in the watchPosition function is called. | |||
* The following data is passed to the event: | |||
{| class="wikitable" | |||
|- | |||
|location.coords.longitude || The current longitude of the device. GPS required. | |||
|- | |||
|location.coords.latitude || The current latitude of the device. GPS required. | |||
|- | |||
|location.coords.altitude|| The height of the location. GPS required. | |||
|- | |||
|location.coords.accuracy || The accuracy of the location. GPS required. | |||
|- | |||
|location.coords.altitudeAccuracy || The accuracy of the altitude. GPS required. | |||
|- | |||
|location.coords.heading|| Degrees clockwise from North. GPS required. | |||
|- | |||
|location.coords.speed|| Speed, meter per second. GPS required. | |||
|- | |||
|location.coords.timestamp || time and date of the observation. GPS required. | |||
|} | |||
* Here's the code to start generating geolocation events: | |||
<pre> | |||
Dim gps | |||
function btnStart_onclick() | |||
options={timeout: 5000, maximumAge: 5000, enableHighAccuracy: True} | |||
gps=navigator.geolocation.watchPosition(onGeolocation, errorCallBack, options) | |||
End function | |||
</pre> | |||
* This code will call onGeolocation every 5 seconds. | |||
* If GPS data cannot be obtained, the errorCallBack function is called. | |||
* Cancel it by executing: | |||
<pre> | |||
navigator.geolocation.clearWatch(gps) | |||
</pre> | |||
* Here's what the onGeolocation looks like. It gets called every 5 seconds. | |||
<pre> | |||
function onGeolocation(location) | |||
Dim s | |||
s = "Longitude: " + location.coords.longitude & vbCRLF | |||
s = s & "Latitude: " + location.coords.latitude & vbCRLF | |||
s = s & "Speed: " + location.coords.speed & " " | |||
s = s & "Altitude: " + location.coords.altitude & vbCRLF | |||
s = s & "Accuracy: " + location.coords.accuracy & " " | |||
s = s & "Accuracy(altitude): " + location.coords.altitudeAccuracy & " " & vbCRLF | |||
'Convert timestamp if needed. | |||
if IsNumeric(location.timestamp) Then | |||
gpsDate=new Date(location.timestamp) | |||
else | |||
gpsDate=location.timestamp | |||
End if | |||
TextArea1.value = s & "Timestamp: " + gpsDate | |||
ShowMap(location.coords.latitude, location.coords.longitude) | |||
End function | |||
</pre> | |||
* This function displays the GPS results in a TextArea. | |||
* The code has to deal with the problem of different browsers returning timestamp in different formats. | |||
* The ShowMap() function near the end displays a map from Google Maps. | |||
<pre> | |||
function ShowMap(latitude, longitude) | |||
if SysInfo(10)-lastRefresh<10000 Then Exit function | |||
lastRefresh=SysInfo(10) | |||
s = "'https://maps.google.com/maps/api/staticmap?center=" & _ | |||
latitude & "," & longitude & _ | |||
"&zoom=14&size=300x200&maptype=roadmap&sensor=true&output=embed'" | |||
Map.innerHTML="<img width=300 height=200 src=" & s & "></img>" | |||
End function | |||
</pre> | |||
* This function displays a Google map. | |||
* The map is updated every 10 seconds | |||
* More info on Google maps here: http://code.google.com/apis/maps/documentation/staticmaps/ | |||
* We pack the latitude and longitude into a URL query string. | |||
* We display the results of the URL in an HTMLview. | |||
= Accelerometer = | = Accelerometer = |
Revision as of 23:24, 10 December 2013
Compass
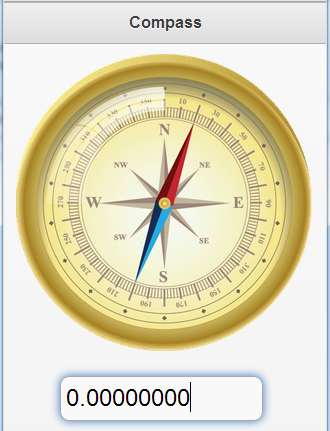
- Certain devices have a built in compass sensor.
- If they do, they call the ondeviceorientation() function when the compass reading changes.
- An event object is passed to the function with the compass reading in evt.webkitCompassHeading.
function window_ondeviceorientation(evt) TextBox1.value=evt.webkitCompassHeading End function
- The Compass sample uses this information to rotate an image of a compass.
- The webKitTransform function rotates an image efficiently.
pos="0" function window_ondeviceorientation(e) imgCompass.style.webkitTransform="rotate(" & pos & "deg)" pos = e.webkitCompassHeading imgCompass.style.webkitTransform="rotate(-" & pos & "deg)" End function
Geolocation
- Geolocation uses GPS sensors to return the current location of the device.
- The watchPosition() function sets the frequency of the Geolocation events.
- When a Geolocation event is triggered, the function named in the watchPosition function is called.
- The following data is passed to the event:
location.coords.longitude | The current longitude of the device. GPS required. |
location.coords.latitude | The current latitude of the device. GPS required. |
location.coords.altitude | The height of the location. GPS required. |
location.coords.accuracy | The accuracy of the location. GPS required. |
location.coords.altitudeAccuracy | The accuracy of the altitude. GPS required. |
location.coords.heading | Degrees clockwise from North. GPS required. |
location.coords.speed | Speed, meter per second. GPS required. |
location.coords.timestamp | time and date of the observation. GPS required. |
- Here's the code to start generating geolocation events:
Dim gps function btnStart_onclick() options={timeout: 5000, maximumAge: 5000, enableHighAccuracy: True} gps=navigator.geolocation.watchPosition(onGeolocation, errorCallBack, options) End function
- This code will call onGeolocation every 5 seconds.
- If GPS data cannot be obtained, the errorCallBack function is called.
- Cancel it by executing:
navigator.geolocation.clearWatch(gps)
- Here's what the onGeolocation looks like. It gets called every 5 seconds.
function onGeolocation(location) Dim s s = "Longitude: " + location.coords.longitude & vbCRLF s = s & "Latitude: " + location.coords.latitude & vbCRLF s = s & "Speed: " + location.coords.speed & " " s = s & "Altitude: " + location.coords.altitude & vbCRLF s = s & "Accuracy: " + location.coords.accuracy & " " s = s & "Accuracy(altitude): " + location.coords.altitudeAccuracy & " " & vbCRLF 'Convert timestamp if needed. if IsNumeric(location.timestamp) Then gpsDate=new Date(location.timestamp) else gpsDate=location.timestamp End if TextArea1.value = s & "Timestamp: " + gpsDate ShowMap(location.coords.latitude, location.coords.longitude) End function
- This function displays the GPS results in a TextArea.
- The code has to deal with the problem of different browsers returning timestamp in different formats.
- The ShowMap() function near the end displays a map from Google Maps.
function ShowMap(latitude, longitude) if SysInfo(10)-lastRefresh<10000 Then Exit function lastRefresh=SysInfo(10) s = "'https://maps.google.com/maps/api/staticmap?center=" & _ latitude & "," & longitude & _ "&zoom=14&size=300x200&maptype=roadmap&sensor=true&output=embed'" Map.innerHTML="<img width=300 height=200 src=" & s & "></img>" End function
- This function displays a Google map.
- The map is updated every 10 seconds
- More info on Google maps here: http://code.google.com/apis/maps/documentation/staticmaps/
- We pack the latitude and longitude into a URL query string.
- We display the results of the URL in an HTMLview.